반응형
문제
심해에는 두 종류의 생명체 A와 B가 존재한다. A는 B를 먹는다. A는 자기보다 크기가 작은 먹이만 먹을 수 있다. 예를 들어, A의 크기가 {8, 1, 7, 3, 1}이고, B의 크기가 {3, 6, 1}인 경우에 A가 B를 먹을 수 있는 쌍의 개수는 7가지가 있다. 8-3, 8-6, 8-1, 7-3, 7-6, 7-1, 3-1.
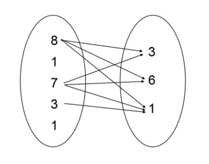
두 생명체 A와 B의 크기가 주어졌을 때, A의 크기가 B보다 큰 쌍이 몇 개나 있는지 구하는 프로그램을 작성하시오.
입력
첫째 줄에 테스트 케이스의 개수 T가 주어진다. 각 테스트 케이스의 첫째 줄에는 A의 수 N과 B의 수 M이 주어진다. 둘째 줄에는 A의 크기가 모두 주어지며, 셋째 줄에는 B의 크기가 모두 주어진다. 크기는 양의 정수이다. (1 ≤ N, M ≤ 20,000)
출력
각 테스트 케이스마다, A가 B보다 큰 쌍의 개수를 출력한다.
예제 입력 1
2
5 3
8 1 7 3 1
3 6 1
3 4
2 13 7
103 11 290 215
예제 출력 1
7
1
소스코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Arrays;
import java.util.StringTokenizer;
public class Main {
private static BufferedReader bf;
private static StringTokenizer st;
private static int T, N, M;
private static int []A, B;
public static void main(String[] args) throws NumberFormatException, IOException {
bf=new BufferedReader(new InputStreamReader(System.in));
T=Integer.parseInt(bf.readLine());
for(int t=1;t<=T;t++) {
st=new StringTokenizer(bf.readLine());
N=Integer.parseInt(st.nextToken());
M=Integer.parseInt(st.nextToken());
A=new int[N];
B=new int[M];
st=new StringTokenizer(bf.readLine());
for(int i=0;i<N;i++) A[i]=Integer.parseInt(st.nextToken());
Arrays.sort(A);
st=new StringTokenizer(bf.readLine());
for(int i=0;i<M;i++) B[i]=Integer.parseInt(st.nextToken());
Arrays.sort(B);
int result=0;
for(int i=0;i<N;i++) {
int cnt=0;
int start=0;
int end=M-1;
while(start<=end) {
int mid=(start+end)/2;
if(A[i]>B[mid]) { // A깂이 B보다 클 때의 (B의 인덱스+1)이 A보다 작은 B값의 개수이므로 이 값을 저장해줍니다.
cnt=mid+1;
start=mid+1;
} else {
end=mid-1;
}
}
result+=cnt;
}
System.out.println(result);
}
}
}
반응형
'코딩테스트 > 이진 탐색(Binary Search)' 카테고리의 다른 글
[Java] 백준 2343번 : 기타 레슨 (0) | 2022.03.27 |
---|---|
[Java] 백준 1072번 : 게임 (0) | 2022.03.25 |
[Python] 백준 11662번 : 민호와 강호 (0) | 2022.01.13 |
[Python] 백준 2110번 : 공유기 설치 (0) | 2022.01.12 |
[Python] 백준 2805번 : 나무 자르기 (0) | 2022.01.09 |