반응형
문제
체스판 위에 한 나이트가 놓여져 있다. 나이트가 한 번에 이동할 수 있는 칸은 아래 그림에 나와있다. 나이트가 이동하려고 하는 칸이 주어진다. 나이트는 몇 번 움직이면 이 칸으로 이동할 수 있을까?
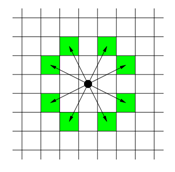
입력
입력의 첫째 줄에는 테스트 케이스의 개수가 주어진다.
각 테스트 케이스는 세 줄로 이루어져 있다. 첫째 줄에는 체스판의 한 변의 길이 l(4 ≤ l ≤ 300)이 주어진다. 체스판의 크기는 l × l이다. 체스판의 각 칸은 두 수의 쌍 {0, ..., l-1} × {0, ..., l-1}로 나타낼 수 있다. 둘째 줄과 셋째 줄에는 나이트가 현재 있는 칸, 나이트가 이동하려고 하는 칸이 주어진다.
출력
각 테스트 케이스마다 나이트가 최소 몇 번만에 이동할 수 있는지 출력한다.
예제 입력 1
3
8
0 0
7 0
100
0 0
30 50
10
1 1
1 1
예제 출력 1
5
28
0
소스코드
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.LinkedList;
import java.util.Queue;
import java.util.StringTokenizer;
public class Main {
private static BufferedReader bf;
private static StringTokenizer st;
private static int T, L, srcX, srcY, destX, destY;
private static int[] dx = { -1, -2, -2, -1, 1, 2, 2, 1 };
private static int[] dy = { -2, -1, 1, 2, 2, 1, -1, -2 };
private static Night night;
private static int [][] map;
static class Night {
int r, c;
public Night(int r, int c) {
super();
this.r = r;
this.c = c;
}
@Override
public String toString() {
return "Night [r=" + r + ", c=" + c + "]";
}
}
public static void main(String[] args) throws NumberFormatException, IOException {
bf = new BufferedReader(new InputStreamReader(System.in));
T = Integer.parseInt(bf.readLine());
for (int t = 1; t <= T; t++) {
L = Integer.parseInt(bf.readLine());
map=new int[L][L];
st = new StringTokenizer(bf.readLine());
srcX = Integer.parseInt(st.nextToken());
srcY = Integer.parseInt(st.nextToken());
night=new Night(srcX, srcY);
st = new StringTokenizer(bf.readLine());
destX = Integer.parseInt(st.nextToken());
destY = Integer.parseInt(st.nextToken());
bfs(night);
System.out.println(map[destX][destY]);
}
}
public static void bfs(Night night) {
Queue<Night> queue=new LinkedList<>();
queue.offer(night);
boolean visited[][] = new boolean[L][L];
visited[night.r][night.c]=true;
while(!queue.isEmpty()) {
Night n=queue.poll();
for(int i=0;i<8;i++) {
int nx=n.r+dx[i];
int ny=n.c+dy[i];
if(isIn(nx, ny) && !visited[nx][ny]) {
visited[nx][ny]=true;
map[nx][ny]=map[n.r][n.c]+1;
if(nx==destX && ny==destY) return;
queue.offer(new Night(nx, ny));
}
}
}
}
public static boolean isIn(int r, int c) {
return r >= 0 && r < L && c >= 0 && c < L;
}
}
bfs를 통해 시작점과 목표지점이 동일해질 때까지 탐색합니다.
반응형
'코딩테스트 > DFS & BFS' 카테고리의 다른 글
[Java] 백준 2589번 : 보물섬 (0) | 2022.03.04 |
---|---|
[Java] 백준 18405번 : 경쟁적 전염 (0) | 2022.03.04 |
[Java] 백준 16236번 : 아기 상어 (0) | 2022.03.02 |
[Java] 백준 7569번 : 토마토 (0) | 2022.03.01 |
[Java] 백준 7576번 : 토마토 (0) | 2022.02.25 |